SQL Data Types Explained: A Complete Guide to Database Types
Unlock the mysteries of SQL data types with our comprehensive guide, designed to enhance your database skills.
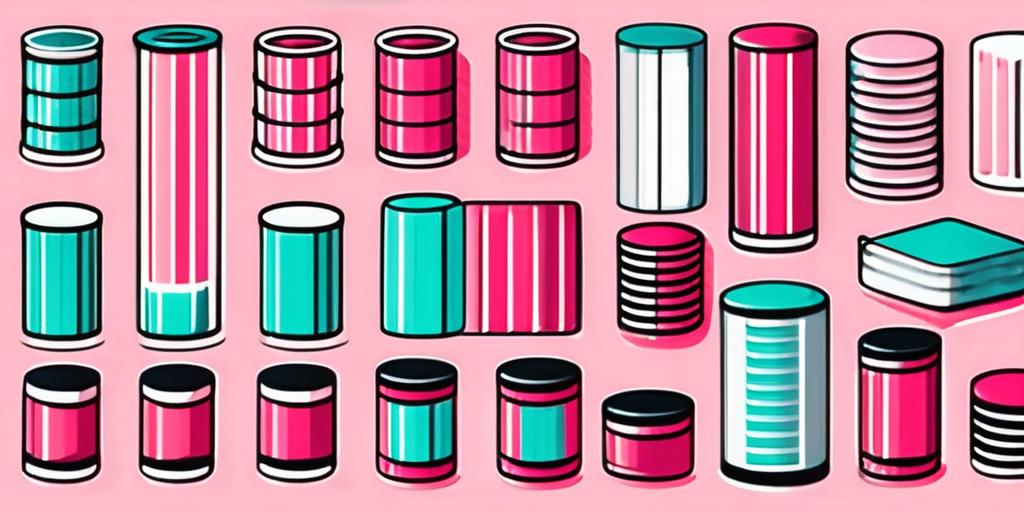
Understanding SQL Data Types
SQL data types define the kind of data that can be stored in a database column. They are fundamental components of SQL and play a crucial role in the structure, integrity, and performance of databases. Each data type serves a specific purpose and is chosen based on the nature of the data being managed.
SQL is a powerful language for managing relational databases, and understanding its data types allows developers and database administrators to create efficient and high-performing applications. Whether the data involved is numeric, textual, or date-related, the right data type helps ensure that the database operates correctly and efficiently.
Definition of SQL Data Types
Data types in SQL are defined by their characteristics and the types of values they can hold. Each SQL database management system (DBMS) may offer slightly different data types, but they generally include categories such as integers, decimals, characters, dates, and binary types. The definition of each data type involves considerations such as the value range, storage requirements, and the operations that can be performed on the data.
For instance, integer types can store whole numbers while decimal types can represent fractional numbers. Character types can hold text strings of varying lengths, and binary types can contain raw binary data. Understanding these definitions is key to effective database design and data manipulation.
Importance of SQL Data Types in Database Management
Choosing the correct SQL data type is essential for numerous reasons. Firstly, the right types minimize storage requirements, which can lead to cost savings, especially in large databases. Secondly, data types help ensure data integrity by enforcing rules around what can be stored in each column. Finally, the correct data types enhance query performance by optimizing how data is processed and retrieved.
Moreover, SQL data types influence how data is validated and transformed throughout the database lifecycle. Understanding their importance can guide developers in creating more robust and reliable data structures that enhance application performance. For example, using the appropriate character data type can prevent issues such as truncation or overflow, which can lead to data loss or corruption. Additionally, employing date and time data types correctly can facilitate accurate time-based queries, which are crucial for applications that rely on historical data analysis or scheduling functionalities.
Furthermore, the choice of data types can also affect indexing strategies. When a column is indexed, the type of data it holds can influence the efficiency of the index and the speed of query execution. For instance, indexing a column with appropriately sized integer data types can significantly improve performance compared to using larger, less efficient types. This highlights the interconnectedness of data types with other database features, emphasizing the need for careful consideration during the design phase to ensure optimal performance and scalability of the database system.Comprehensive Overview of SQL Data Types
SQL data types can be broadly classified into several categories. Each category serves a specific type of data and is suited to various application requirements. Below is an overview of the main categories of SQL data types.
Numeric Data Types in SQL
Numeric data types are used to store numbers. They include integers (whole numbers) and floating-point numbers (numbers with fractional parts). Common numeric types include:
- INT: A standard integer type that can vary in size
- DECIMAL(p, s): A fixed-point number, where 'p' is precision, and 's' is scale
- FLOAT: A floating-point number that allows for a larger range of values
Choosing between these types typically depends on the required precision and range of values. For instance, use DECIMAL for financial calculations where precision is critical, while FLOAT is useful for scientific measurements where some precision loss is acceptable. Additionally, understanding the limits of each numeric type is crucial; for example, INT can typically store values from -2,147,483,648 to 2,147,483,647, while DECIMAL can handle much larger numbers with defined precision, making it suitable for applications like banking systems where exact figures are mandatory.
Date and Time Data Types in SQL
Date and time data types are designed to store timestamps, dates, and intervals. The most commonly used types include:
- DATE: Stores a date value in YYYY-MM-DD format
- TIME: Stores a time value in HH:MM:SS format
- DATETIME: Combines both date and time in a single type
Using these data types correctly ensures that date-related calculations and comparisons are accurate, which is vital for any application that manages temporal data. Moreover, SQL also provides types like TIMESTAMP, which records the date and time of an event with time zone awareness, making it essential for applications that operate across multiple time zones. This capability is particularly important for logging events in distributed systems, where understanding the exact timing of actions is critical for troubleshooting and analysis.
String (Character and Binary) Data Types in SQL
SQL provides various data types for storing strings of text, which can include characters and binary data. Common types include:
- CHAR(n): A fixed-length character string
- VARCHAR(n): A variable-length character string, where 'n' defines the maximum size
- BINARY: Stores binary data with fixed length
Using string data types efficiently can significantly impact the performance of SQL queries. When selecting string types, consider how the application will utilize text data, including length requirements and character set considerations. For instance, VARCHAR is often preferred for user-generated content, as it can save space by only using the necessary amount of storage, while CHAR might be more suitable for fixed-length codes like postal codes or country codes, where the length is consistent across entries.
Unicode Character String Data Types
With the increasing globalization of applications, support for multiple languages has become essential. Unicode data types allow for the storage of characters from various languages and scripts. Important types include:
- NCHAR(n): Fixed-length Unicode character string
- NVARCHAR(n): Variable-length Unicode character string
These Unicode types are crucial for supporting international applications and ensuring text is correctly stored and displayed regardless of language. Furthermore, using NVARCHAR can be particularly advantageous in applications where text data can vary significantly in length and language, as it allows for more efficient storage and retrieval of multilingual content. This flexibility is vital for modern applications that cater to diverse user bases, ensuring that all users can interact with the system in their preferred language.
Miscellaneous SQL Data Types
Aside from the primary data types, SQL also includes miscellaneous types that serve specific purposes. Some examples are:
- JSON: Stores JSON (JavaScript Object Notation) data
- XML: Stores XML (eXtensible Markup Language) data
- ENUM: Represents a string object that can have one value from a set of predefined values
These types are beneficial for applications that require structured and semi-structured data management and manipulation. For instance, the JSON data type allows developers to store complex data structures directly in a SQL database, facilitating easier integration with web applications that utilize JSON for data interchange. Similarly, XML data types are advantageous for applications that need to handle hierarchical data, such as configuration files or data feeds from external systems. ENUM types can simplify data validation by restricting values to a defined set, which can enhance data integrity and reduce errors in user input.
```
Choosing the Right SQL Data Type
Selecting the appropriate SQL data type involves careful consideration of various factors that can impact the functionality and efficiency of the database. An informed choice is crucial for optimizing performance and reducing potential data issues.
Factors to Consider When Selecting Data Types
When choosing a data type, several key factors should be evaluated:
- Data Size: Estimate the data size to choose the most efficient type
- Data Range: Understand the possible values to select a type that can accommodate all required data
- Query Performance: Consider how different data types may affect the speed of queries
By taking these factors into account, developers can design databases that are not only functional but also scalable and efficient.
Common Mistakes in Choosing Data Types
Several mistakes can occur during data type selection that may lead to inefficiencies or data integrity issues. Common errors include:
- Choosing a data type that is too large for the data being stored, wasting space
- Using a data type that does not enforce data constraints, leading to invalid entries
- Neglecting potential future data requirements, resulting in costly data migrations
Being aware of these pitfalls can help database designers avoid problems that could hinder application performance or lead to data corruption.
Converting SQL Data Types
Conversions between different SQL data types are a routine requirement in database management. Whether due to changes in application requirements or simply data manipulation needs, understanding conversion processes is key to maintaining data integrity.
Implicit and Explicit Conversion
SQL supports two types of data conversion: implicit and explicit. Implicit conversion occurs automatically when SQL server needs to convert one data type to another, usually without any loss of data. For instance, converting an integer to a floating-point number may happen seamlessly.
On the other hand, explicit conversion requires the developer to specify the conversion method. This is done using conversion functions such as CAST() or CONVERT(). Explicit conversion is important when precision or data loss becomes a concern and the developer needs specific control over the conversion process.
Conversion Functions in SQL
SQL offers several built-in functions for converting types. The primary functions are:
- CAST(expression AS data_type): Converts an expression to the specified data type
- CONVERT(data_type, expression): Similar to CAST, with additional formatting options
Using these conversion functions allows developers to manage their data more effectively and ensures that data retains its intended meaning and structure throughout its lifecycle.
In conclusion, understanding SQL data types is fundamental for anyone looking to work effectively with relational databases. By grasping how to choose, use, and convert these data types, developers can create robust, efficient, and reliable database systems that meet their application requirements.
Now that you're equipped with a comprehensive understanding of SQL data types and their pivotal role in database management, it's time to elevate your data governance and analytics capabilities with CastorDoc. Embrace the power of a user-friendly AI assistant integrated with advanced governance tools to streamline your data operations. Whether you're a data professional seeking to maintain impeccable data quality and compliance or a business user aiming to harness data for strategic insights, CastorDoc is your partner in unlocking the full potential of your data assets. Don't miss the opportunity to transform your data management experience. Try CastorDoc today and witness a revolution in data accessibility and analytics.
You might also like
Get in Touch to Learn More



“[I like] The easy to use interface and the speed of finding the relevant assets that you're looking for in your database. I also really enjoy the score given to each table, [which] lets you prioritize the results of your queries by how often certain data is used.” - Michal P., Head of Data